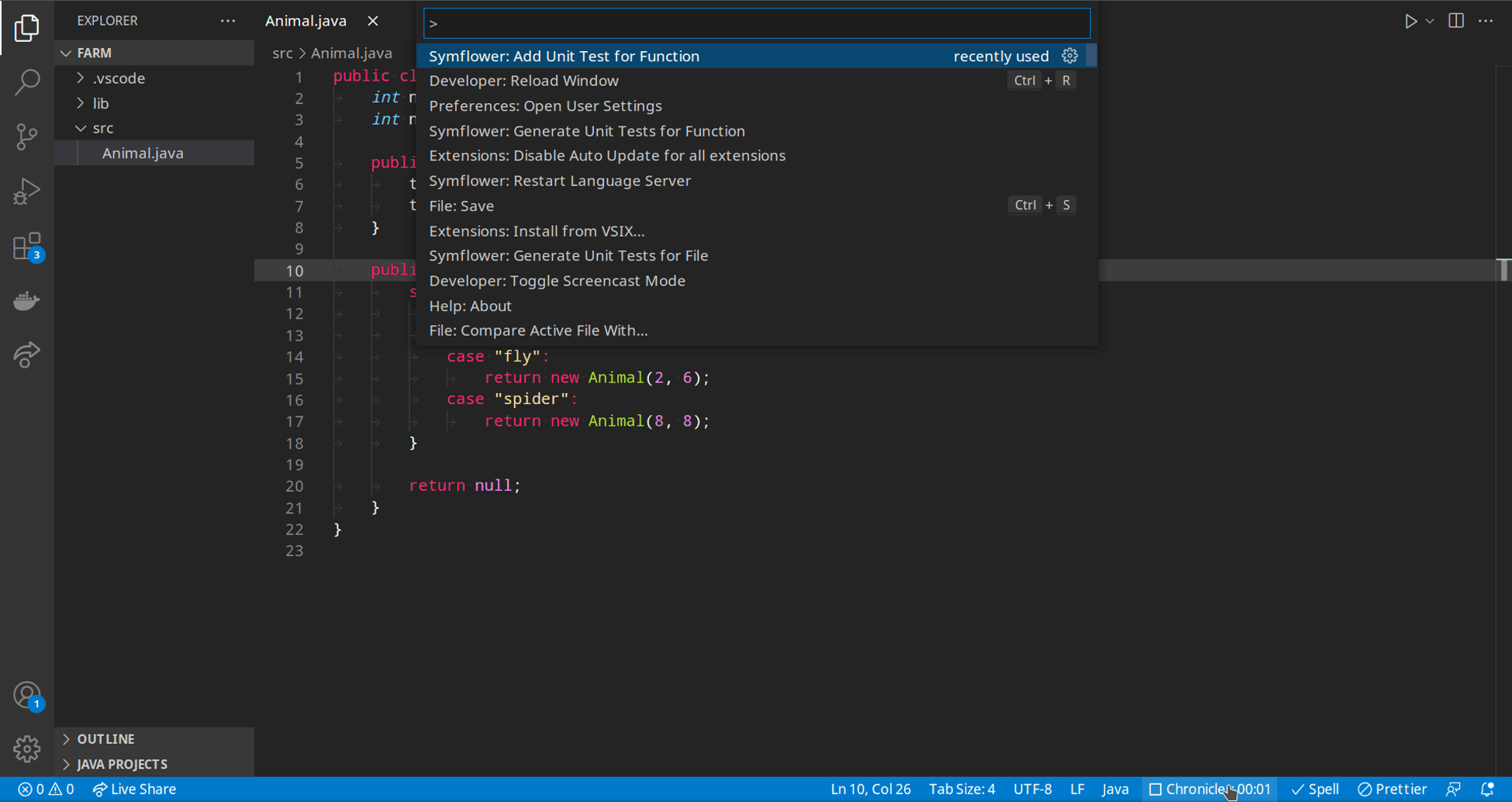
Unit tests are not just a nice-to-have, but a must-have in most projects. They let you focus on your implementation as the existing behavior can be freezed with a good unit test suite, that is, there will be no surprises when you change the implementation.
However, there is probably a small part in every developer’s brain which thinks “unit tests, oh no, not again”. At Symflower, we want to help you to silence this voice in your head by equipping you with the best tools possible for hassle-free unit testing. That’s why we added the feature of generating Java test templates to our Visual Studio Code extension so you can also add manually written tests faster and without effort.
In case you are short on a piece of code to try it out, we’ve got you covered:
public class Animal {
int numberOfEyes;
int numberOfLegs;
public Animal(int eyes, int legs) {
this.numberOfEyes = eyes;
this.numberOfLegs = legs;
}
public static Animal fromString(String animal) {
switch (animal) {
case "cat":
return new Animal(2, 4);
case "fly":
return new Animal(2, 6);
case "spider":
return new Animal(8, 8);
}
return null;
}
}
By opening the context menu and clicking on the entry “Symflower: Add unit test for Function” (or executing the same-named VS Code command), a test file is automatically created if it does not yet exist, opened and a test function is appended for the code you want to test. Best of all, your cursor is immediately set right where you need it, so you can start completing your test.
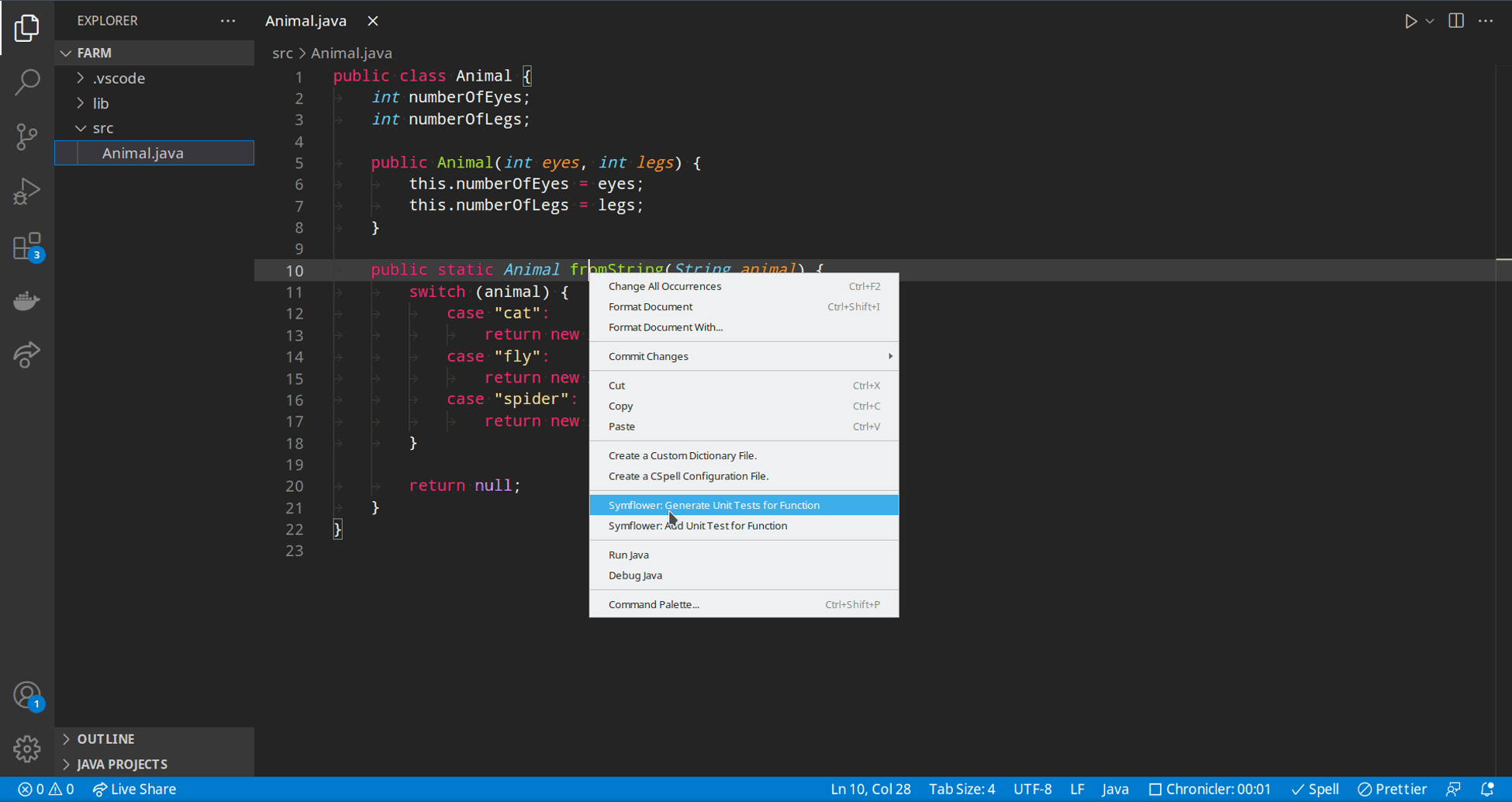
In case you want to completely silence your inner anti-unit-test voice, you can even let Symflower generate complete tests for you, including actual test values. Check out our tutorial to see it in action! Or just execute the command “Symflower: Generate Unit Tests for Function” in the above example.
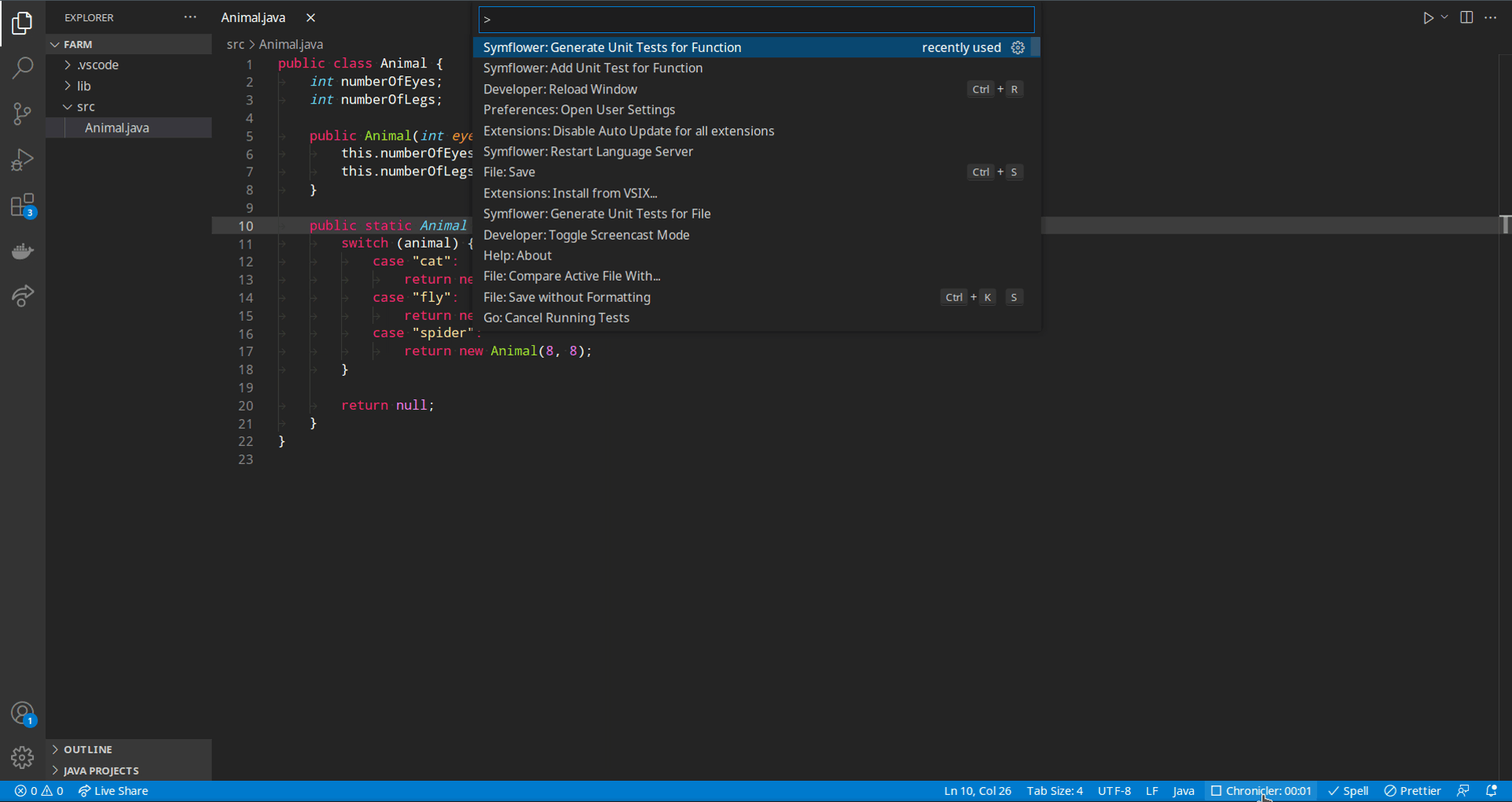
For the multilingual developers: Both features, unit test templates and the generation of complete unit tests, are already supported for Go. Checkout our blog post about Better table-driven testing in Go to find out more!
We’d love to hear back from you how you like the Java test templates. If you feel like something is missing or if you found a bug, please let us know via Twitter, LinkedIn or our public issue tracker.
Don’t forget to subscribe to our newsletter and follow us on Twitter, LinkedIn, and Facebook for getting notified on new articles about software development and software infrastructure, or if you are simply into memes.